はじめに
今回はSpringBatchの起動方法をまとめました。
筆者の環境を簡単に記述します。
- Eclipse 2022
- MySQL 8.0
- JavaSE 17
- ビルド -> gradle
- 依存関係 -> SpringBatch , MySQL Driver
SpringBatchを解説
- 流れとしては、まずはバッチの処理の起点となる「コンフィグクラス」を作成します。
- Taskletでビジネスロジックを定義します。
- 上記で定義したロジックを実行するための環境を設定します。
※JobとStep
バッチ設定のコンフィグクラスを作成する
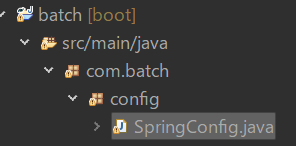
SpringConfig
package com.batch.config;
import org.springframework.batch.core.launch.JobLauncher;
import org.springframework.batch.core.repository.JobRepository;
import org.springframework.context.annotation.Configuration;
import org.springframework.transaction.PlatformTransactionManager;
/**
* 設定クラス
* このクラスはアプリケーションの設定を管理します。
*/
@Configuration
public class SpringConfig {
private final JobLauncher jobLauncher;
private final JobRepository jobRepository;
private final PlatformTransactionManager platformTransactionManager;
public SpringConfig(JobLauncher jobLauncher, JobRepository jobRepository,
PlatformTransactionManager platformTransactionManager) {
this.jobLauncher = jobLauncher;
this.jobRepository = jobRepository;
this.platformTransactionManager = platformTransactionManager;
}
}
Tasklet
Taskletとは、Step内で実行される処理を記述するためのインターフェースです。
「execute()」メソッドを実装する事でビジネスロジックを定義する事が出来ます。
以下、例です。
・ファイルの読み込み
・データベース更新
・メールの送信
それでは、以下のようにjavaファイルを作成しましょう。
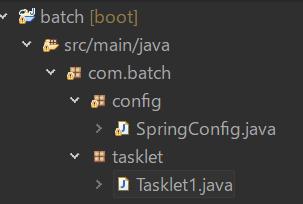
Tasklet1
package com.batch.tasklet;
import org.springframework.batch.core.StepContribution;
import org.springframework.batch.core.configuration.annotation.StepScope;
import org.springframework.batch.core.scope.context.ChunkContext;
import org.springframework.batch.core.step.tasklet.Tasklet;
import org.springframework.batch.repeat.RepeatStatus;
import org.springframework.stereotype.Component;
@Component("Tasklet1")
@StepScope
public class Tasklet1 implements Tasklet{
@Override
public RepeatStatus execute(StepContribution contribution, ChunkContext chunkContext) throws Exception {
System.out.println("Tasklet1の出力です");
return RepeatStatus.FINISHED;
}
}
SpringConfigへ定義を挿入します。
SpringConfig
package com.batch.config;
import org.springframework.batch.core.launch.JobLauncher;
import org.springframework.batch.core.repository.JobRepository;
import org.springframework.batch.core.step.tasklet.Tasklet;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Configuration;
import org.springframework.transaction.PlatformTransactionManager;
/**
* 設定クラス
* このクラスはアプリケーションの設定を管理します。
*/
@Configuration
public class SpringConfig {
private final JobLauncher jobLauncher;
private final JobRepository jobRepository;
private final PlatformTransactionManager platformTransactionManager;
@Autowired
@Qualifier("Tasklet1")
private Tasklet Tasklet1;
public SpringConfig(JobLauncher jobLauncher, JobRepository jobRepository,
PlatformTransactionManager platformTransactionManager) {
this.jobLauncher = jobLauncher;
this.jobRepository = jobRepository;
this.platformTransactionManager = platformTransactionManager;
}
}
JobとStep
JobとStepの関係は以下です。
- Jobは、バッチ処理全体を管理し、Stepはその中の個別の処理を実行します。
- Jobは、複数のStepを含む事ができ、Stepは順番に実行されます。
- ステップが終了するごとに、次のステップに進むかどうか、エラーが発生した場合にどうするかなどを制御できます。
「SpringConfig」を以下のように編集します。
SpringConfig
package com.batch.config;
import org.springframework.batch.core.Job;
import org.springframework.batch.core.Step;
import org.springframework.batch.core.job.builder.JobBuilder;
import org.springframework.batch.core.launch.JobLauncher;
import org.springframework.batch.core.launch.support.RunIdIncrementer;
import org.springframework.batch.core.repository.JobRepository;
import org.springframework.batch.core.step.builder.StepBuilder;
import org.springframework.batch.core.step.tasklet.Tasklet;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.transaction.PlatformTransactionManager;
/**
* 設定クラス
* このクラスはアプリケーションの設定を管理します。
*/
@Configuration
public class SpringConfig {
// ジョブの実行を管理する
private final JobLauncher jobLauncher;
// ジョブの実行結果や進行状況をデータベースへ記録する
private final JobRepository jobRepository;
// トランザクションを開始し、コミットまたはロールバックする
private final PlatformTransactionManager platformTransactionManager;
@Autowired
@Qualifier("Tasklet1")
private Tasklet Tasklet1;
public SpringConfig(JobLauncher jobLauncher, JobRepository jobRepository,
PlatformTransactionManager platformTransactionManager) {
this.jobLauncher = jobLauncher;
this.jobRepository = jobRepository;
this.platformTransactionManager = platformTransactionManager;
}
// Stepを定義する
@Bean
public Step tasklet1Step1() {
// 第一引数はStep名を定義する
return new StepBuilder("tasklet1Step1" , jobRepository)
// Taskletを指定する
.tasklet(Tasklet1 , platformTransactionManager)
.build();
}
// Jobを定義する
@Bean
public Job tasklet1Job1() {
// 第一引数はJob名を定義する
return new JobBuilder("tasklet1Job1" , jobRepository)
// ジョブを実行するたびに一意のIDを生成
.incrementer(new RunIdIncrementer())
// 実行するStepを指定する
.start(tasklet1Step1())
.build();
}
}
Spring Batchを実行する
ここまで記述していくと、Spring Batchを実行する事ができます。実行した内容はデータベースへ格納されるため、現在作業されている環境にデータベースのインストールが無い方はインストールする必要があるのでご注意ください。
筆者はお馴染みのMySQLを使用します。この記述はエクリプスとMySQLを連携するための設定記述と覚えておきましょう!
「application.properties」に以下を記述します。
Java
spring.datasource.url=jdbc:mysql://${MYSQL_HOST:localhost}:3306/batchDB?createDatabaseIfNotExist=true
spring.datasource.username=root
spring.datasource.password=ご自身のパスワード
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.sql.init.encoding=utf-8
spring.batch.jdbc.initialize-schema = ALWAYS
以下の解説です。
batchDB?createDatabaseIfNotExist=true
batchDBという名前のデータベースが無ければ、自動で作成してください。という意味です。
それでは、実行します。今回のバッチを実行すると「Tasklet1の出力です」が出力されれば成功ですね。
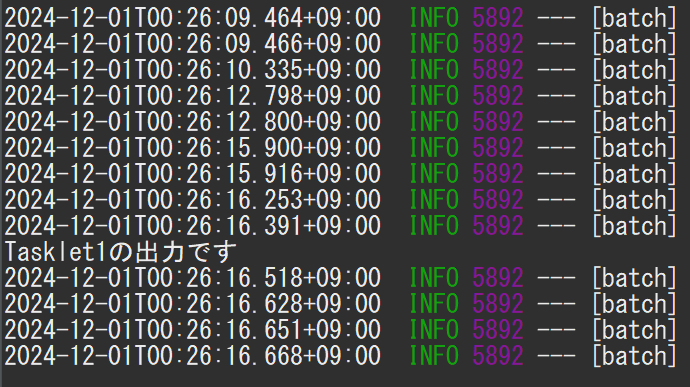
出力されましたね!成功です。それでは、DBも見に行ってみましょう!
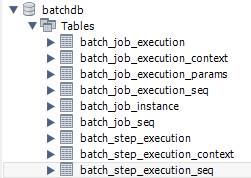
自動でデータベースと、jobとstepのテーブルを作成してくれました。
おわりに
かなり初歩の内容だとは思いますが、少しでも役に立てれば幸いです。
コメント